Today we are looking at how to create calender in php using JQuery and Ajax,this tutorials is have a web calendar displaying on our web calendar automatically.The feature of this calendar is that it has a left and right arrow which can use to navigate to next and previous month and year.For those of us who may want to test it on a local server before installing it to their web page,you need to run it through a server like Xampp server,Wamp server etc.
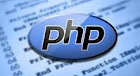
Before we proceed there are some basic things we need to know to make the feature more effectively.
• We need to build a style for our our calendar
• We need a JQuery script to run this feature,you can download your JQuery Script online
• We need to build an Ajax page that will call our PHP class
• We need to build a PHP class
• Finally we also need to create an index page
Steps To Create Our Web Calendar
2. Create a PHP Class and name it class.calender.php with the code given below.
3. Next proceed by creating PHP Ajax page and name it calendar-ajax.php with the below code
4. Finally create an index.php page with the given code below
Note:You have to put all this code in the same folder,also note that the line (35,36,37) which is highlighted is calling the JQuery script I ask you to download earlier before we proceeded to this tutorial.
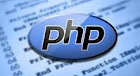
Before we proceed there are some basic things we need to know to make the feature more effectively.
• We need to build a style for our our calendar
• We need a JQuery script to run this feature,you can download your JQuery Script online
• We need to build an Ajax page that will call our PHP class
• We need to build a PHP class
• Finally we also need to create an index page
Steps To Create Our Web Calendar
- Create a page and name it style.css with the below codes
body { font-family: calibri; } #calendar-outer { width: 574px; } #calendar-outer ul { margin: 0px; padding: 0px; } #calendar-outer ul li { margin: 0px; padding: 0px; list-style-type: none; } .prev { display: inline-block; float: left; cursor: pointer } .next { display: inline-block; float: right; cursor: pointer } :focus { outline: none; background: #ff8e8e; } div.calendar-nav { background-color: #ff8e8e; border-radius: 4px; text-align: center; padding: 10px; color: #FFF; box-sizing: border-box; font-weight: bold; } #calendar-outer .week-name-title li { display: inline-block; padding: 10px 27px; color: #90918b; font-size: 0.95em; font-weight: 600; } .week-day-cell li { display: inline-block; width: 80px; height: 80px; text-align: center; line-height: 80px; vertical-align: middle; background-color: #f6ffc6; color: #ff8e8e; border: 1px solid #f1f0f0; border-radius: 4px; font-size: 1.2em; } #body-overlay {background-color: rgba(0, 0, 0, 0.6);z-index: 999;position: absolute;left: 0;top: 0;width: 100%;height: 100%;display: none;} #body-overlay div {position:absolute;left:50%;top:50%;margin-top:-32px;margin-left:-32px;}
2. Create a PHP Class and name it class.calender.php with the code given below.
<?php class PHPCalendar { private $weekDayName = array ("MON","TUE","WED","THU","FRI","SAT","SUN"); private $currentDay = 0; private $currentMonth = 0; private $currentYear = 0; private $currentMonthStart = null; private $currentMonthDaysLength = null; function __construct() { $this->currentYear = date ( "Y", time () ); $this->currentMonth = date ( "m", time () ); if (! empty ( $_POST ['year'] )) { $this->currentYear = $_POST ['year']; } if (! empty ( $_POST ['month'] )) { $this->currentMonth = $_POST ['month']; } $this->currentMonthStart = $this->currentYear . '-' . $this->currentMonth . '-01'; $this->currentMonthDaysLength = date ( 't', strtotime ( $this->currentMonthStart ) ); } function getCalendarHTML() { $calendarHTML = '<div id="calendar-outer">'; $calendarHTML .= '<div class="calendar-nav">' . $this->getCalendarNavigation() . '</div>'; $calendarHTML .= '<ul class="week-name-title">' . $this->getWeekDayName () . '</ul>'; $calendarHTML .= '<ul class="week-day-cell">' . $this->getWeekDays () . '</ul>'; $calendarHTML .= '</div>'; return $calendarHTML; } function getCalendarNavigation() { $prevMonthYear = date ( 'm,Y', strtotime ( $this->currentMonthStart. ' -1 Month' ) ); $prevMonthYearArray = explode(",",$prevMonthYear); $nextMonthYear = date ( 'm,Y', strtotime ( $this->currentMonthStart . ' +1 Month' ) ); $nextMonthYearArray = explode(",",$nextMonthYear); $navigationHTML = '<div class="prev" data-prev-month="' . $prevMonthYearArray[0] . '" data-prev-year = "' . $prevMonthYearArray[1]. '"><</div>'; $navigationHTML .= '<span id="currentMonth">' . date ( 'M', strtotime ( $this->currentMonthStart ) ) . '</span>'; $navigationHTML .= '<span contenteditable="true" id="currentYear" style="margin-left:5px">'. date ( 'Y', strtotime ( $this->currentMonthStart ) ) . '</span>'; $navigationHTML .= '<div class="next" data-next-month="' . $nextMonthYearArray[0] . '" data-next-year = "' . $nextMonthYearArray[1]. '">></div>'; return $navigationHTML; } function getWeekDayName() { $WeekDayName= ''; foreach ( $this->weekDayName as $dayname ) { $WeekDayName.= '<li>' . $dayname . '</li>'; } return $WeekDayName; } function getWeekDays() { $weekLength = $this->getWeekLengthByMonth (); $firstDayOfTheWeek = date ( 'N', strtotime ( $this->currentMonthStart ) ); $weekDays = ""; for($i = 0; $i < $weekLength; $i ++) { for($j = 1; $j <= 7; $j ++) { $cellIndex = $i * 7 + $j; $cellValue = null; if ($cellIndex == $firstDayOfTheWeek) { $this->currentDay = 1; } if (! empty ( $this->currentDay ) && $this->currentDay <= $this->currentMonthDaysLength) { $cellValue = $this->currentDay; $this->currentDay ++; } $weekDays .= '<li>' . $cellValue . '</li>'; } } return $weekDays; } function getWeekLengthByMonth() { $weekLength = intval ( $this->currentMonthDaysLength / 7 ); if($this->currentMonthDaysLength % 7 > 0) { $weekLength++; } $monthStartDay= date ( 'N', strtotime ( $this->currentMonthStart) ); $monthEndingDay= date ( 'N', strtotime ( $this->currentYear . '-' . $this->currentMonth . '-' . $this->currentMonthDaysLength) ); if ($monthEndingDay < $monthStartDay) { $weekLength++; } return $weekLength; } } ?>
3. Next proceed by creating PHP Ajax page and name it calendar-ajax.php with the below code
<?php require_once 'class.calendar.php'; $phpCalendar = new PHPCalendar (); $calendarHTML = $phpCalendar->getCalendarHTML(); echo $calendarHTML; ?>
4. Finally create an index.php page with the given code below
<?php require_once 'class.calendar.php'; $phpCalendar = new PHPCalendar (); ?> <html> <head> <link href="style.css" type="text/css" rel="stylesheet" /> <title>PHP Calendar</title> </head> <body> <div id="body-overlay"><div><img src="loading.gif" width="64px" height="64px"/></div></div> <div id="calendar-html-output"> <?php $calendarHTML = $phpCalendar->getCalendarHTML(); echo $calendarHTML; ?> </div> <script src="jquery-1.11.2.min.js" type="text/javascript"></script> <script> $(document).ready(function(){ $(document).on("click", '.prev', function(event) { var month = $(this).data("prev-month"); var year = $(this).data("prev-year"); getCalendar(month,year); }); $(document).on("click", '.next', function(event) { var month = $(this).data("next-month"); var year = $(this).data("next-year"); getCalendar(month,year); }); $(document).on("blur", '#currentYear', function(event) { var month = $('#currentMonth').text(); var year = $('#currentYear').text(); getCalendar(month,year); }); }); function getCalendar(month,year){ $("#body-overlay").show(); $.ajax({ url: "calendar-ajax.php", type: "POST", data:'month='+month+'&year='+year, success: function(response){ setInterval(function() {$("#body-overlay").hide(); },500); $("#calendar-html-output").html(response); }, error: function(){} }); } </script> </body> </html>
Note:You have to put all this code in the same folder,also note that the line (35,36,37) which is highlighted is calling the JQuery script I ask you to download earlier before we proceeded to this tutorial.
Get our updates delivered to your inbox
No comments:
Post a Comment